This example shows how to change the Cursor of the mouse pointer for a specific element and for the application.This example consists of a Extensible Application Markup Language (XAML) file and a code behind file.
<Windowx:Class="WpfApplication1.Window1"xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"Title="cursors"Height="450" Width="600" Loaded="OnLoaded">
<Window.Resources>
<Style Target Type="{x:Type Radio Button}">
<Setter Property="Margin" Value="3" />
</Style>
<Style TargetType="{x:Type Label}">
<Setter Property="FontSize" Value="14" />
<Setter Property="HorizontalAlignment" Value="Center" />
</Style>
<Style TargetType="{x:Type Border}">
<Setter Property="BorderBrush" Value="LightBlue" />
<Setter Property="BorderThickness" Value="2" />
<Setter Property="Margin" Value="10" />
</Style>
</Window.Resources>
<StackPanel>
<Border Width="300">
<StackPanel Orientation="Horizontal" HorizontalAlignment="Center">
<Label HorizontalAlignment="Left">Cursor Type</Label>
<ComboBox Width="100" SelectionChanged="CursorTypeChanged"HorizontalAlignment="Left" Name="CursorSelector">
<ComboBoxItem Content="AppStarting" />
<ComboBoxItem Content="ArrowCD" />
<ComboBoxItem Content="Arrow" />
<ComboBoxItem Content="Cross" />
<ComboBoxItem Content="HandCursor" />
<ComboBoxItem Content="Help" />
<ComboBoxItem Content="IBeam" />
<ComboBoxItem Content="No" />
<ComboBoxItem Content="None" />
<ComboBoxItem Content="Pen" />
<ComboBoxItem Content="ScrollSE" />
<ComboBoxItem Content="ScrollWE" />
<ComboBoxItem Content="SizeAll" />
<ComboBoxItem Content="SizeNESW" />
<ComboBoxItem Content="SizeNS" />
<ComboBoxItem Content="SizeNWSE" />
<ComboBoxItem Content="SizeWE" />
<ComboBoxItem Content="UpArrow" />
<ComboBoxItem Content="WaitCursor" />
<ComboBoxItem Content="Custom" />
</ComboBox>
</StackPanel>
</Border>
<Border Name="DisplayArea" Height="250" Width="400" Margin="20" Background="AliceBlue">
<Label HorizontalAlignment="Center">Move Mouse Pointer Over This Area</Label>
</Border>
</StackPanel>
</Window>
// code behind the File:Window.xaml.vb
Imports System
Imports System.Windows
Imports System.Windows.Controls
Imports System.Windows.Media
Imports System.Windows.Input
Imports System.IO
Imports System.Collections
Namespace WpfApplication1
Partial Public Class Window1
Inherits Window
Private CustomCursor As Cursor
Public Sub CursorTypeChanged(ByVal sender As Object, ByVal e AsSelectionChangedEventArgs)
Dim source As ComboBox = TryCast(e.Source, ComboBox)
If source IsNot Nothing Then
Dim selectedCursor As ComboBoxItem = TryCast(source.SelectedItem, ComboBoxItem)
Select Case selectedCursor.Content.ToString()
Case "AppStarting"
DisplayArea.Cursor = Cursors.AppStarting
Exit Select
Case "ArrowCD"
DisplayArea.Cursor = Cursors.ArrowCD
Exit Select
Case "Arrow"
DisplayArea.Cursor = Cursors.Arrow
Exit Select
Case "Cross"
DisplayArea.Cursor = Cursors.Cross
Exit Select
Case "HandCursor"
DisplayArea.Cursor = Cursors.Hand
Exit Select
Case "Help"
DisplayArea.Cursor = Cursors.Help
Exit Select
Case "IBeam"
DisplayArea.Cursor = Cursors.IBeam
Exit Select
Case "No"
DisplayArea.Cursor = Cursors.No
Exit Select
Case "None"
DisplayArea.Cursor = Cursors.None
Exit Select
Case "Pen"
DisplayArea.Cursor = Cursors.Pen
Exit Select
Case "ScrollSE"
DisplayArea.Cursor = Cursors.ScrollSE
Exit Select
Case "ScrollWE"
DisplayArea.Cursor = Cursors.ScrollWE
Exit Select
Case "SizeAll"
DisplayArea.Cursor = Cursors.SizeAll
Exit Select
Case "SizeNESW"
DisplayArea.Cursor = Cursors.SizeNESW
Exit Select
Case "SizeNS"
DisplayArea.Cursor = Cursors.SizeNS
Exit Select
Case "SizeNWSE"
DisplayArea.Cursor = Cursors.SizeNWSE
Exit Select
Case "SizeWE"
DisplayArea.Cursor = Cursors.SizeWE
Exit Select
Case "UpArrow"
DisplayArea.Cursor = Cursors.UpArrow
Exit Select
Case "WaitCursor"
DisplayArea.Cursor = Cursors.Wait
Exit Select
Case "Custom"
DisplayArea.Cursor = CustomCursor
Exit Select
Case Else
Exit Select
End Select
End If
End Sub
Public Sub OnLoaded(ByVal sender As Object, ByVal e As RoutedEventArgs)
DirectCast(CursorSelector.Items(0), ComboBoxItem).IsSelected = True
End Sub
End Class
End Namespace
This is simplest way that how to use cursor in Window Presentation Form.
OUTPUT OF THE ABOVE APPLICATION
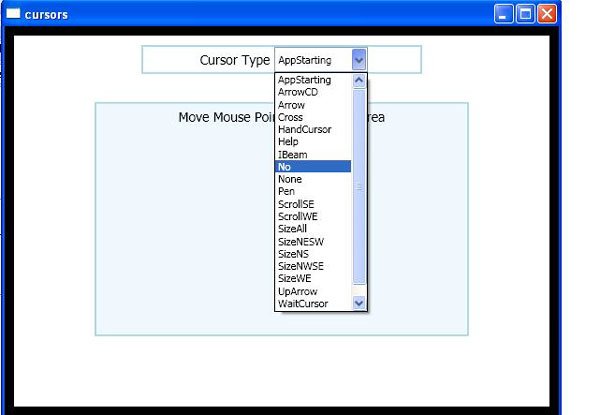