JQuery Tooltip Plugins in ASP.NET using VB.NET
This topic introduces the JQuery tooltips and explain how to create and customize tooltip content in JQuery.
JQuery Tooltip
A tooltip allows you to easily show a fancy pop-up window that appears when a user's mouse hovers over an element which you designate in your web page. Here I will show you how you to create a simple tooltip using JQuery.
Code Snippets
<html>
<head>
<title>Example</title>
<script type="text/javascript" src="jquery.js"></script>
<style type="text/css">
#hint
{
cursor:pointer;
}
.tooltip
{
margin:8px;
padding:8px;
border:1px solid blue;
background-color:yellow;
position: absolute;
z-index: 2;
}
.style1
{
color: #FF5050;
}
.style2
{
font-size: medium;
}
</style>
</head>
<body>
<form id="form1" runat="server" style="background-color: #FFFFFF">
<h1 style="border-style: groove; font-family: Verdana; height: 31px; background-color: #EEEEEE;"
class="style2">JQuery ToolTips</h1>
<hr style="color: #000000; height: -15px;">
<p style="font-family: Verdana; font-size: small; background-color: #EEEEEE;">Full Name:
<asp:TextBox ID="TextBox1" runat="server" Width="164px"></asp:TextBox>
<span class="style1"><strong style="background-color: #EEEEEE">(MouseOver me to view Tooltip)</strong></span></p>
<p style="font-family: Verdana; font-size: small; background-color: #EEEEEE;">Address:
<asp:TextBox ID="TextBox2" runat="server" Width="164px"></asp:TextBox>
</p>
<p style="font-family: Verdana; font-size: small; background-color: #EEEEEE;">Date Of Birth:
<asp:TextBox ID="TextBox3" runat="server" Width="164px"></asp:TextBox>
</p>
<p style="font-family: Verdana; font-size: small; background-color: #EEEEEE;">Qualifications:
<asp:TextBox ID="TextBox4" runat="server" Width="164px"></asp:TextBox>
</p>
<p style="font-family: Verdana; font-size: small; background-color: #EEEEEE;">Contact No:
<asp:TextBox ID="TextBox5" runat="server" Width="164px"></asp:TextBox>
</p>
<p style="font-family: Verdana; font-size: small">
<asp:Button ID="Button1" runat="server" Font-Names="Verdana" Font-Size="Small"
Height="28px" style="font-weight: 700" Text="Submit" />
</p>
<script type="text/javascript">
$(document).ready(function () {
var changeTooltipPosition = function (event) {
var tooltipX = event.pageX - 8;
var tooltipY = event.pageY + 8;
$('div.tooltip').css({ top: tooltipY, left: tooltipX });
};
var showTooltip = function (event) {
$('div.tooltip').remove();
$('<div class="tooltip">I\' am tooltips! tooltips! tooltips! :)</div>')
.appendTo('body');
changeTooltipPosition(event);
};
var hideTooltip = function () {
$('div.tooltip').remove();
};
$("span#hint,label#username'").bind({
mousemove: changeTooltipPosition,
mouseenter: showTooltip,
mouseleave: hideTooltip
});
});
</script></form>
</body>
</html>
Output Result
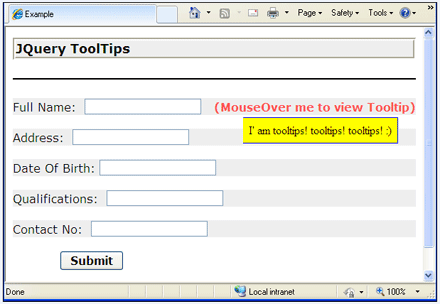
I hope this will help you.