ComboBox Items
The Items property is used to add and work with items in a ComboBox. We can add items to a ComboBox at design-time from Properties Window by clicking on Items Collection as you can see in Figure 5.
Figure 5
When you click on the Collections, the String Collection Editor window will pop up where you can type strings. Each line added to this collection will become a ComboBox item. I add four items as you can see from Figure 6.
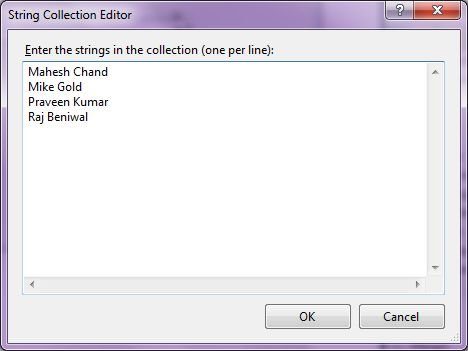
Figure 6
The ComboBox looks like Figure 7.
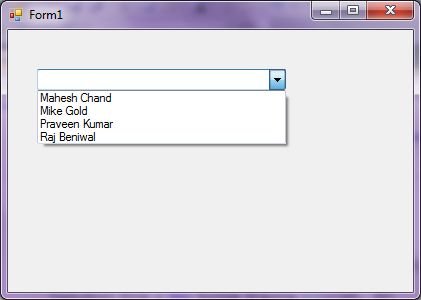
Figure 7
You can add same items at run-time by using the following code snippet.
ComboBox1.Items.Add("Mahesh Chand")
ComboBox1.Items.Add("Mike Gold")
ComboBox1.Items.Add("Praveen Kumar")
ComboBox1.Items.Add("Raj Beniwal")
Getting All Items
To get all items, we use the Items property and loop through it to read all the items. The following code snippet loops through all items and adds item contents to a StringBuilder and displays in a MessageBox.
Dim sb As New System.Text.StringBuilder
For Each item In ComboBox1.Items
sb.Append(item)
sb.Append(" ")
Next
MessageBox.Show(sb.ToString())
Selected Text and Item
Text property is used to set and get text of a ComboBox. The following code snippet sets and gets current text of a ComboBox.
ComboBox1.Text = "Mahesh Chand"
MessageBox.Show(ComboBox1.Text)
We can also get text associated with currently selected item by using Items property.
Dim selectedItem As String = ComboBox1.Items(ComboBox1.SelectedIndex)
Why the value of ComboBox.SelectedText is Empty?
SelectedText property gets and sets the selected text in a ComboBox only when a ComboBox has focus on it. If the focus moves away from a ComboBox, the value of SelectedText will be an empty string. To get current text in a ComboBox when it does not have focus, use Text property.
DataSource
DataSource property is used to get and set a data source to a ComboBox. The data source can be a collection or object that implements IList interface such as an array, a collection, or a DataSet. The following code snippet binds an enumeration converted to an array to a ComboBox.
ComboBox1.DataSource = System.Enum.GetValues(GetType(ComboBoxStyle))
DropDownStyle
DropDownStyle property is used to gets and sets the style of a ComboBox. It is a type of ComboBoxStyle enumeration.
The ComboBoxStyle enumeration has following three values.
· Simple - List is always visible and the text portion is editable.
· DropDown â€" List is displayed by clicking the down arrow and that the text portion is editable.
· DropDownList - List is displayed by clicking the down arrow and that the text portion is not editable.
The following code snippet sets the DropDownStyle property of a ComboBox to DropDownList.
ComboBox1.DropDownStyle = ComboBoxStyle.DropDownList
DroppedDown
If set true, the dropped down portion of the ComboBox is displayed. By default, this value is false.
Sorting Items
The Sorted property set to true, the ComboBox items are sorted. The following code snippet sorts the ComboBox items.
ComboBox1.Sorted = True
Find Items
The FindString method is used to find a string or substring in a ComboBox. The following code snippet finds a string in a ComboBox and selects it if found.
Private Sub FindStringButton_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles FindStringButton.Click
Dim index As Integer = ComboBox1.FindString(TextBox1.Text)
If (index < 0) Then
MessageBox.Show("Item not found.")
TextBox1.Text = String.Empty
Else
ComboBox1.SelectedIndex = index
End If
End Sub