A VScrollBar control is a supporting control that is used to add vertical scrolling capability to a control that does not have built-in scrolling such as a container control. You do not need this control for the controls that already have built-in scrolling.
In this article, I will discuss how to create a VScrollBar control and apply vertical scrolling on Windows Forms controls that do not have built-in scrolling feature. After that, I will discuss various properties and methods available for the VScrollBar control.
Creating a VScrollBar
We can create a VScrollBar control using a Forms designer at design-time or using the VScrollBar class in code at run-time (also known as dynamically).
To create a VScrollBar control at design-time, you simply drag and drop a VScrollBar control from Toolbox to a Form in Visual Studio. After you drag and drop a VScrollBar on a Form, the VScrollBar looks like Figure 1. Once a VScrollBar is on the Form, you can move it around and resize it using mouse and set its properties and events.
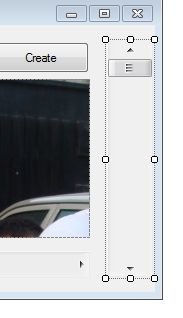
Figure 1
Creating a VScrollBar control at run-time is merely a work of creating an instance of VScrollBar class, set its properties and add VScrollBar class to the Form controls.
First step to create a dynamic VScrollBar is to create an instance of VScrollBar class. The following code snippet creates a VScrollBar control object.
Dim vScroller As New VScrollBar()
In the next step, you may set properties of a VScrollBar control. The following code snippet sets the height and width properties of a VScrollBar.
vScroller.Height = 200
vScroller.Width = 30
Once a VScrollBar control is ready with its properties, next step is to add the VScrollBar control to the Form. To do so, we use Form.Controls.Add method. The following code snippet adds a VScrollBar control to the current Form.
Me.Controls.Add(vScroller)
Setting VScrollBar Properties
After you place a VScrollBar control on a Form, the next step is to set properties.
The easiest way to set properties is from the Properties Window. You can open Properties window by pressing F4 or right click on a control and select Properties menu item. The Properties window looks like Figure 2.
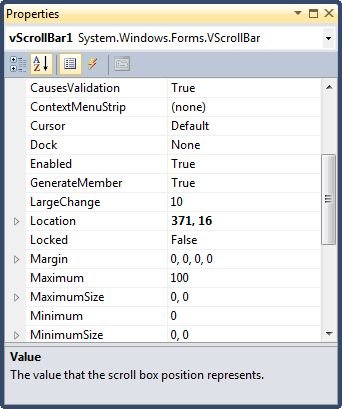
Figure 2
Location, Height, Width, and Size
The Location property takes a Point that specifies the starting position of the VScrollBar on a Form. The Size property specifies the size of the control. We can also use Width and Height property instead of Size property. The Dock property is used to dock a control. The following code snippet sets Dock, Width, and Height properties of a VScrollBar control.
vScroller.Dock = DockStyle.Right
vScroller.Width = 30
vScroller.Height = 200
Name
Name property represents a unique name of a VScrollBar control. It is used to access the control in the code. The following code snippet sets and gets the name and text of a VScrollBar control.
authorGroup.Name = "VScrollBar1"
Maximum, Minimum, and Value
Value property of a VScrollBar represents the current value of a VScrollBar control. The Minimum and Maximum properties are used to set minimum and maximum limit of a VScrollBar. The following code snippet sets the Minimum, Maximum, and Value properties of a VScrollBar control.
vScroller.Minimum = 0
vScroller.Maximum = 100
vScroller.Value = 40
Attaching VScrollBar to a Control
Horizontal scroll bar control is attached to a control by its scroll event. On the scroll event hander, we usually read the current value of a VScrollBar and based on this value, we apply on other controls. For example, we can implement scrolling in a PictureBox control by displaying image in a PictureBox again on the scroll event bar.
The following code snippet adds an event handler for the Scroll event.
Friend WithEvents vScroller As New VScrollBar
The following code snippet is used to redraw the image on a PictureBox based on the value of the VScrollBar.
Private Sub vScroller_Scroll(ByVal sender As System.Object, _
ByVal e As System.Windows.Forms.ScrollEventArgs) _
Handles vScroller.Scroll
Dim g As Graphics = PictureBox1.CreateGraphics()
g.DrawImage(PictureBox1.Image,
New Rectangle(0, 0, PictureBox1.Height, vScroller.Value))
End Sub
Summary
A VScrollBar control is used to add vertical scrolling feature to a Windows Forms control that do not have built-in scrolling feature. In this article, we discussed discuss how to create a VScrollBar control in Windows Forms at design-time as well as run-time. After that, we saw how to use various properties and methods.