A LinkLabel control is a label control that can display a hyperlink. A LinkLabel control is inherited from the Label class so it has all the functionality provided by the Windows Forms Label control. LinkLabel control does not participate in user input or capture mouse or keyboard events.
In this article, I will discuss how to create a LinkLabel control in Windows Forms at design-time as well as run-time. After that, I will continue discussing various properties and methods available for the LinkLabel control.
In Visual Studio 2010, the ToolStripLabel control is recommended for a LinkLabel control.
Creating a LinkLabel
There are two ways to create a control.
Design-time
First, we can use the Form designer of Visual Studio to create a control at design-time. In design-time mode, we can use visual user interfaces to create a control properties and write methods.
To create a LinkLabel control at design-time, you simply drag and drop a LinkLabel control from Toolbox to a Form. After you drag and drop a LinkLabel on a Form. The LinkLabel looks like Figure 1. Once a LinkLabel is on the Form, you can move it around and resize it using mouse and set its properties and events.
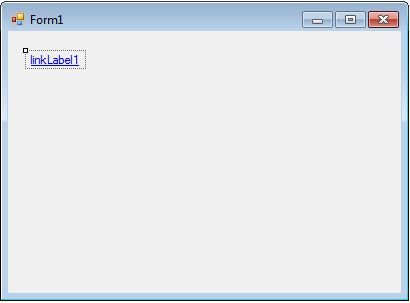
Figure 1
Run-time
LinkLabel class represents a hyperlink Label control. We simply create an instance of LinkLabel class, set its properties and add this it to the Form controls.
In the first step, we create an instance of the LinkLabel class. The following code snippet creates a LinkLabel control object.
Dim dynamicLinkLabel As New LinkLabel
In the next step, we set properties of a LinkLabel control. The following code snippet sets background color, foreground color, Text, Name, and Font properties of a LinkLabel.
dynamicLinkLabel.BackColor = Color.Red
dynamicLinkLabel.ForeColor = Color.Blue
dynamicLinkLabel.Text = "I am a Dynamic LinkLabel"
dynamicLinkLabel.Name = "DynamicLinkLabel"
dynamicLinkLabel.Font = New Font("Georgia", 16)
In the last step, we need to add a LinkLabel control to the Form by calling Form.Controls.Add method. The following code snippet adds a LinkLabel control to a Form.
Controls.Add(dynamicLinkLabel)
Setting LinkLabel Properties
After you place a LinkLabel control on a Form, the next step is to set properties.
The easiest way to set properties is from the Properties Window. You can open Properties window by pressing F4 or right click on a control and select Properties menu item. The Properties window looks like Figure 2.
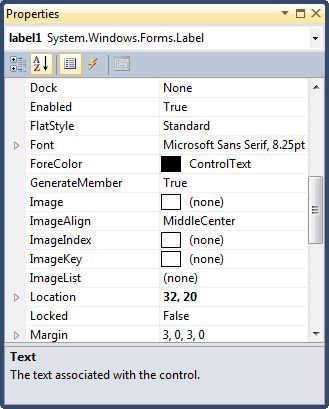
Figure 2
Name
Name property represents a unique name of a LinkLabel control. It is used to access the control in the code. The following code snippet sets and gets the name and text of a LinkLabel control.
dynamicLinkLabel.Name = "DynamicLinkLabel"
Location, Height, Width, and Size
The Location property takes a Point that specifies the starting position of the LinkLabel on a Form. The Size property specifies the size of the control. We can also use Width and Height property instead of Size property. The following code snippet sets Location, Width, and Height properties of a LinkLabel control.
dynamicLinkLabel.Location = New Point(20, 150)
dynamicLinkLabel.Height = 40
dynamicLinkLabel.Width = 300
Background, Foreground, BorderStyle
BackColor and ForeColor properties are used to set background and foreground color of a LinkLabel respectively. If you click on these properties in Properties window, the Color Dialog pops up.
Alternatively, you can set background and foreground colors at run-time. The following code snippet sets BackColor and ForeColor properties.
dynamicLinkLabel.BackColor = Color.Red
dynamicLinkLabel.ForeColor = Color.Blue
You can also set borders style of a LinkLabel by using the BorderStyle property. The BorderStyle property is represented by a BorderStyle enumeration that has three values â€" FixedSingle, Fixed3D, and None. The default value of border style is Fixed3D. The following code snippet sets the border style of a LinkLabel to FixedSingle.
dynamicLinkLabel.BorderStyle = BorderStyle.FixedSingle
Font
Font property represents the font of text of a LinkLabel control. If you click on the Font property in Properties window, you will see Font name, size and other font options. The following code snippet sets Font property at run-time.
dynamicLinkLabel.Font = New Font("Georgia", 16)
Text and TextAlign, and TextLength
Text property of a LinkLabel represents the current text of a LinkLabel control. The TextAlign property represents text alignment that can be Left, Center, or Right. The TextLength property returns the length of a LinkLabel contents.
The following code snippet sets the Text and TextAlign properties and gets the size of a LinkLabel control.
dynamicLinkLabel.Text = "I am Dynamic LinkLabel"
dynamicLinkLabel.TextAlign = HorizontalAlignment.Center
Append Text
We can append text to a LinkLabel by simply setting Text property to current text plus new text you would want to append something like this.
dynamicLinkLabel.Text += " Appended text"
AutoEllipsis
An ellipsis character (...) is used to give an impression that a control has more characters but it could not fit in the current width of the control. If AutoEllipsis property is true, it adds ellipsis character to a control if text in control does not fit. You may have to set AutoSize to false to see the ellipses character.
Image in LinkLabel
The Image property of a LinkLabel control is used to set a LinkLabel background as an image. The Image property needs an Image object. The Image class has a static method called FromFile that takes an image file name with full path and creates an Image object.
You can also align image and text. The ImageAlign and TextAlign properties of Button are used for this purpose.
The following C# code snippet sets an image as a LinkLabel background.
dynamicLinkLabel.Image = Image.FromFile(@"C:\Images\Dock.jpg")
dynamicLinkLabel.ImageAlign = ContentAlignment.MiddleRight
dynamicLinkLabel.TextAlign = ContentAlignment.MiddleLeft
dynamicLinkLabel.FlatStyle = FlatStyle.Flat
Hyperlink Properties
Here are the hyperlink related properties available in the LinkLabel control.
Links and LinkArea
A LinkLabel control can display more than one hyperlink. The Links property a type of LinkCollection represents all the hyperlinks available in a LinkLabel control. The Add method of LinkColleciton is used to add a link to the collection. The Remove and RemoveAt methods are used to remove a link from the LinkCollection. The Clear method is used to remove all links from a LinkCollection.
LinkArea property represents the range of text that is treated as a part of the link. It takes a starting position and length of the text.
The following code snippet ads a link and sets LinkArea and a link click event handler.
dynamicLinkLabel.LinkArea = new LinkArea(0, 22)
dynamicLabel.Links.Add(24, 9, "http://www.c-sharpcorner.com")
dynamicLabel.LinkClicked += new LinkLabelLinkClickedEventHandler(LinkedLabelClicked);
Here is the code for the LinkLabel click event handler and uses Process.Start method to open a hyperlink in a browser.
private void LinkedLabelClicked(object sender, LinkLabelLinkClickedEventArgs e)
{
dynamicLabel.LinkVisited = true;
System.Diagnostics.Process.Start("http://www.c-sharpcorner.com");
}
LinkColor, VisitedLinkColor, ActiveLinkColor and DisabledLinkColor
LinkColor, VisitedLinkColor, ActiveLinkColor and DisabledLinkColor properties represent colors when a hyperlink is in normal, visited, active, or disabled mode. The following code snippet sets these colors.
dynamicLabel.ActiveLinkColor = Color.Orange;
dynamicLabel.VisitedLinkColor = Color.Green;
dynamicLabel.LinkColor = Color.RoyalBlue;
dynamicLabel.DisabledLinkColor = Color.Gray;
Summary
A LinkLabel control is used to display a hyperlink and can be used to click the hyperlink to open a URL.. In this article, we discussed discuss how to create a LinkLabel control in Windows Forms at design-time as well as run-time. After that, we saw how to use various properties and methods.