A ServiceController component allows us to access and manage Windows Services running on a machine. In this article, I will discuss how to a ServiceController class to load all services and get information about them in a Windows Forms application using Visual Studio 2010.
Adding System.ServiceProcess Reference
A ServiceController represents a Windows Service and defined in the System.ServiceProcess namespace. Before this namespace can be imported, you must add reference to the System.ServiceProcess assembly.
To add reference to an assembly, right click on the project name in Visual Studio and select Add Reference menu item and then browse the assembly you need to add to your application.
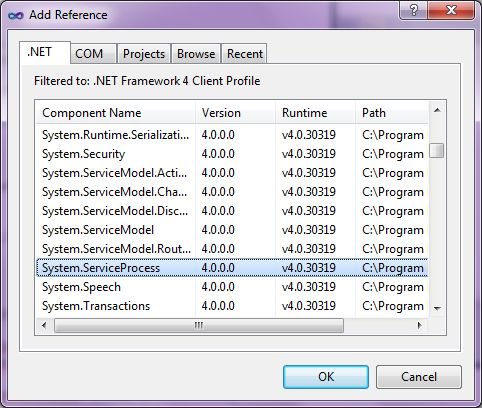
Getting all services installed on a machine
ServiceController.GetServices static method returns list of all services running on a machine. The following code snippet gets all the services and displays them in a ListBox.
For Each service As ServiceController In ServiceController.GetServices()
Dim serviceName As String = service.ServiceName
ListBox1.Items.Add(serviceName)
Next
ServiceController Properties
Here is a list of ServiceController class properties.
-
CanPauseAndContinue - Service can be paused and continued.
-
CanShutdown - Service can be shut down.
-
CanStop - Service can be stopped.
-
DisplayName - Friendly name of a service.
-
MachineName - Name of the computer where this service is installed.
-
ServiceName - Service name.
-
ServiceHandle - Service handle or Id.
-
ServiceType - Type of a service such as device driver, process, or kernel.
-
Status - Status of a service such as running, stopped, or paused.
The following code snippet uses some of these properties.
Private Sub GetAllServices()
For Each service As ServiceController In ServiceController.GetServices()
Dim serviceName As String = service.ServiceName
Dim serviceDisplayName As String = service.DisplayName
Dim serviceType As String = service.ServiceType.ToString()
Dim status As String = service.Status.ToString()
ListBox1.Items.Add(serviceName + " " + serviceDisplayName +
serviceType + " " + status)
Next
End Sub
Getting all Device Driver Services installed on a machine
ServiceController.GetDevices static method returns list of all device driver services running on a machine. The following code snippet gets all the device driver services and displays them in a ListBox.
Private Sub GetAllDevices()
For Each service As ServiceController In ServiceController.GetDevices()
Dim serviceName As String = service.ServiceName
Dim serviceDisplayName As String = service.DisplayName
Dim serviceType As String = service.ServiceType.ToString()
Dim status As String = service.Status.ToString()
ListBox1.Items.Add(serviceName + " " + serviceDisplayName +
serviceType + " " + status)
Next
End Sub
Start, Stop, Pause, Continue, Refresh and Close a Service
We can start, stop, pause, continue and refresh a service using Start, Stop, Pause, Continue, and Refresh methods. Close method disconnects this ServiceController instance from the service and frees all the resources that the instance allocated.
The following code snippet checks if a service is stopped, start it; otherwise stop it.
Private Sub StartStop()
Dim service As ServiceController = New ServiceController("MyServiceName")
If ((service.Status.Equals(ServiceControllerStatus.Stopped)) Or
(service.Status.Equals(ServiceControllerStatus.StopPending))) Then
service.Start()
Else
service.Stop()
End If
End Sub
Summary
In this article, we discussed how to use a ServiceController to get access to services available on a machine.