Color Dialog
A ColorDialog control is used to select a color from available colors and also define custom colors. A typical Color Dialog looks like Figure 1 where you can see there is a list of basic solid colors and there is an option to create custom colors.
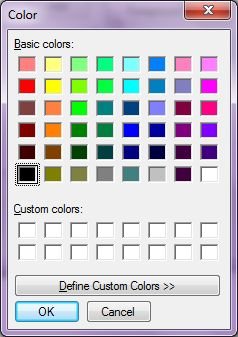
Figure 1
Creating a ColorDialog
We can create a ColorDialog control using a Forms designer at design-time or using the ColorDialog class in code at run-time (also known as dynamically). Unlike other Windows Forms controls, a ColorDialog does not have and not need visual properties like others. The only purpose of ColorDialog to display available colors, create custom colors and select a color from these colors. Once a color is selected, we need that color in our code so we can apply it on other controls.
Again, you can create a ColorDialog at design-time but It is easier to create a ColorDialog at run-time.
Design-time
To create a ColorDialog control at design-time, you simply drag and drop a ColorDialog control from Toolbox to a Form in Visual Studio. After you drag and drop a ColorDialog on a Form, the ColorDialog looks like Figure 2.
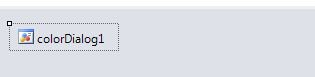
Figure 2
Adding a ColorDialog to a Form adds following two lines of code.
Friend WithEvents ColorDialog1 As System.Windows.Forms.ColorDialog
Me.ColorDialog1 = New System.Windows.Forms.ColorDialog()
Run-time
Creating a ColorDialog control at run-time is merely a work of creating an instance of ColorDialog class, set its properties and add ColorDialog class to the Form controls.
First step to create a dynamic ColorDialog is to create an instance of ColorDialog class. The following code snippet creates a ColorDialog control object.
Dim colorDlg As New ColorDialog()
ShowDialog method of ColorDialog displays the ColorDialog. The following code snippet sets background color, foreground color, Text, Name, and Font properties of a ColorDialog.
colorDlg.ShowDialog()
Once the ShowDialog method is called, you can pick colors on the dialog.
Setting ColorDialog Properties
After you place a ColorDialog control on a Form, the next step is to set properties.
The easiest way to set properties is from the Properties Window. You can open Properties window by pressing F4 or right click on a control and select Properties menu item. The Properties window looks like Figure 3.

Figure 3
AllowFullOpen
If you look at Figure 1, you will see a button called Define Custom Colors on the ColorDialog. Clicking on this button opens the custom color editor area where you can define colors by setting RGB color values (between 0 to 255) and can also select a color from the color area as you can see in Figure 4.
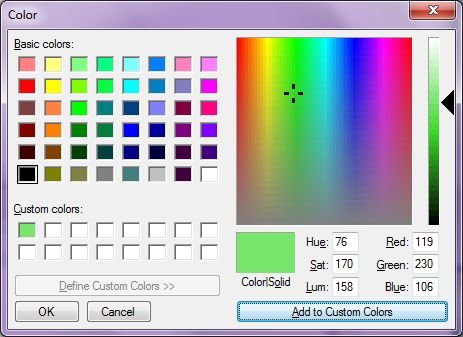
Figure 4
AllowFullOpen property makes sure that Define Custom Color option is enabled on a ColorDialog. If you wish to disable this option, you can set AllowFullOpen property to false and your ColorDialog will look like Figure 5.
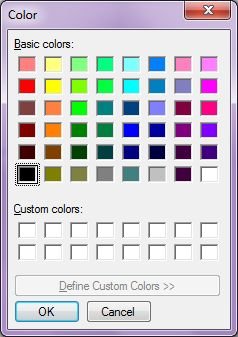
Figure 5
The following code snippet sets the AllowFullOpen property to false.
colorDlg.AllowFullOpen = False
Color, AnyColor, and SolidColorOnly
Color property is used to get and set the color selected by the user in a ColorDialog.
AnyColor is used to get and set whether a ColorDialog displays all available colors in the set of basic colors.
SolidColorOnly is used to get and set whether a ColorDialog restricts users to selecting solid colors only.
The following code snippet sets these properties.
colorDlg.Color = Color.Red
colorDlg.SolidColorOnly = False
Using ColorDialog in Applications
Now let's create an application that will use a ColorDialog to set colors of bunch of controls. The Windows Forms application looks like Figure 6.
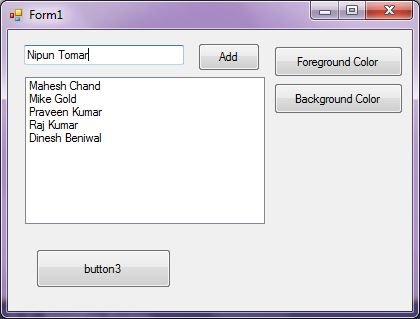
Figure 6
In Figure 6, we have a few Windows Forms controls and clicking on Foreground Color and Background Color buttons will let user select a color and set that color as foreground and background colors of the controls. After selecting foreground and background colors, the Form looks like Figure 7.
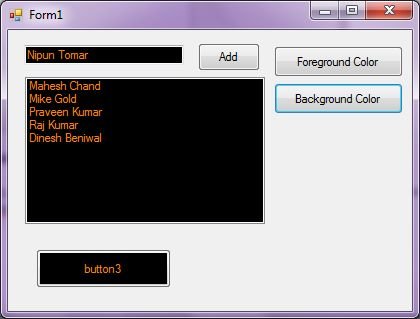
Figure 7
The following code snippet is the code for Foreground Color and Background Color buttons click event handlers.
Public Class Form1
Private Sub ForegroundButton_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles ForegroundButton.Click
Dim colorDlg As New ColorDialog()
colorDlg.AllowFullOpen = False
colorDlg.ShowHelp = True
colorDlg.Color = Color.Red
colorDlg.SolidColorOnly = False
If (colorDlg.ShowDialog() = Windows.Forms.DialogResult.OK) Then
TextBox1.ForeColor = colorDlg.Color
ListBox1.ForeColor = colorDlg.Color
Button3.ForeColor = colorDlg.Color
End If
End Sub
Private Sub BackgroundButton_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles BackgroundButton.Click
Dim colorDlg As New ColorDialog()
If (colorDlg.ShowDialog() = Windows.Forms.DialogResult.OK) Then
TextBox1.BackColor = colorDlg.Color
ListBox1.BackColor = colorDlg.Color
Button3.BackColor = colorDlg.Color
End If
End Sub
Private Sub AddButton_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles AddButton.Click
ListBox1.Items.Add(TextBox1.Text)
End Sub
End Class
Summary
A ColorDialog control allows users to launch Windows Color Dialog and let them select a solid color or create a custom color from available colors. In this article, we discussed how to use a Windows Color Dialog and set its properties in a Windows Forms application.