The FlowLayoutPanel control is a container control that provides dynamically layout for the child controls that can be arranged horizontally or vertically. The flow direction of the control sets the direction of arrangements of controls.
Creating a FlowLayoutPanel
We can create a FlowLayoutPanel control using the Forms designer at design-time or using the FlowLayoutPanel class in code at run-time (also known as dynamically).
Design-time
To create a FlowLayoutPanel control at design-time, you simply drag and drop a FlowLayoutPanel control from Toolbox to a Form in Visual Studio. After you drag and drop a FlowLayoutPanel on a Form, the FlowLayoutPanel looks like Figure 1. Once a FlowLayoutPanel is on the Form, you can move it around and resize it using mouse and set its properties and events.
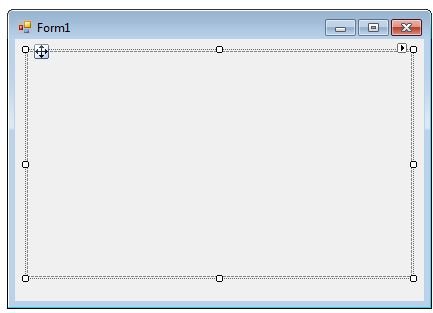
Figure 1
Run-time
Creating a FlowLayoutPanel control at run-time is merely a work of creating an instance of FlowLayoutPanel class, set its properties and adds FlowLayoutPanel class to the Form controls.
First step to create a dynamic FlowLayoutPanel is to create an instance of FlowLayoutPanel class. The following code snippet creates a FlowLayoutPanel control object.
Dim dynamicFlowLayoutPanel As New FlowLayoutPanel
In the next step, you may set properties of a FlowLayoutPanel control. The following code snippet sets location, size and Name properties of a FlowLayoutPanel.
dynamicFlowLayoutPanel.Location = New System.Drawing.Point(26, 12)
dynamicFlowLayoutPanel.Name = "FlowLayoutPanel1"
dynamicFlowLayoutPanel.Size = New System.Drawing.Size(228, 20)
Once the FlowLayoutPanel control is ready with its properties, the next step is to add the FlowLayoutPanel to a Form. To do so, we use Form.Controls.Add method that adds FlowLayoutPanel control to the Form controls and displays on the Form based on the location and size of the control. The following code snippet adds a FlowLayoutPanel control to the current Form.
Me.Controls.Add(dynamicFlowLayoutPanel)
Setting FlowLayoutPanel Properties
After you place a FlowLayoutPanel control on a Form, the next step is to set its properties.
The easiest way to set properties is from the Properties Window. You can open Properties window by pressing F4 or right click on a control and select Properties menu item. The Properties window looks like Figure 2.
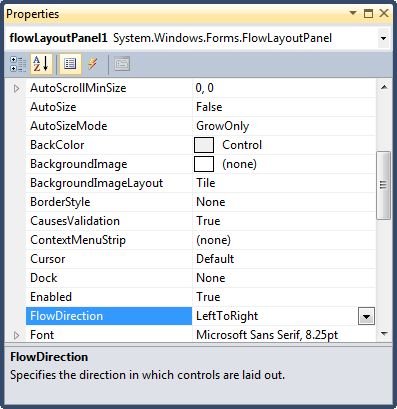
Figure 2
Name
Name property represents a unique name of a FlowLayoutPanel control. It is used to access the control in the code. The following code snippet sets and gets the name and text of a FlowLayoutPanel control.
dynamicFlowLayoutPanel.Name = "FlowLayoutPanel1"
Location, Height, Width and Size
The Location property takes a Point that specifies the starting position of the FlowLayoutPanel on a Form. You may also use Left and Top properties to specify the location of a control from the left top corner of the Form. The Size property specifies the size of the control. We can also use Width and Height property instead of Size property. The following code snippet sets Location, Width, and Height properties of a FlowLayoutPanel control.
dynamicFlowLayoutPanel.Location = New System.Drawing.Point(26, 12)
dynamicFlowLayoutPanel.Size = New System.Drawing.Size(228, 20)
Flow Direction
The FlowDirection property represents the direction in which child controls are arranged on a panel. The FlowDirection property is represented by a FlowDirection enumeration that has four self-explanatory values LeftToRight, TopDown, RightToLeft, and BottomUp values. The following code snippet sets and gets the Value properties at run-time.
dynamicFlowLayoutPanel.FlowDirection = FlowDirection.RightToLeft
OK say we have a Form with a few controls on it arranged on a FlowLayoutPanel as displayed in Figure 3. The default flow direction of a FlowLayoutPanel is LeftToRight. Make sure WrapContents property is set to False.
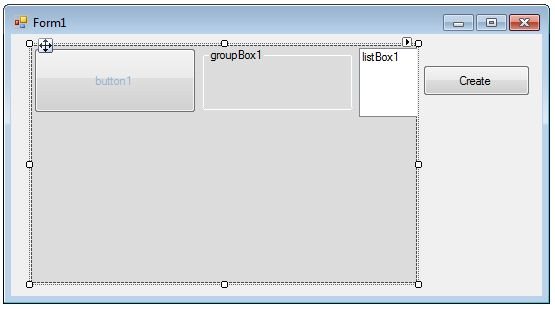
Figure 3
If we change FlowDirection to RightToLeft, new Form looks like Figure 4.
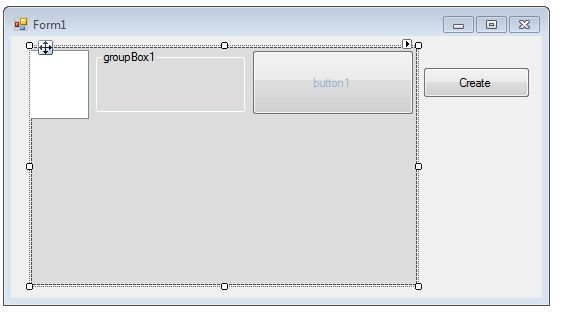
Figure 4
If we change FlowDirection to TopDown, new Form looks like Figure 5.
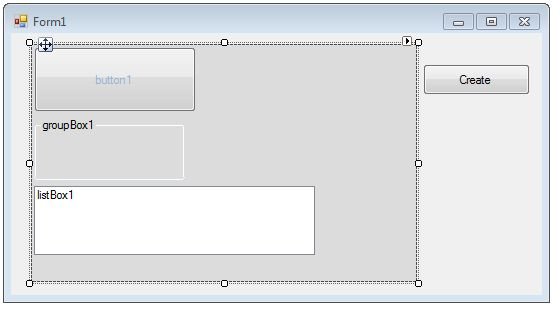
Figure 5
If we change FlowDirection to BottomUp, new Form looks like Figure 6.
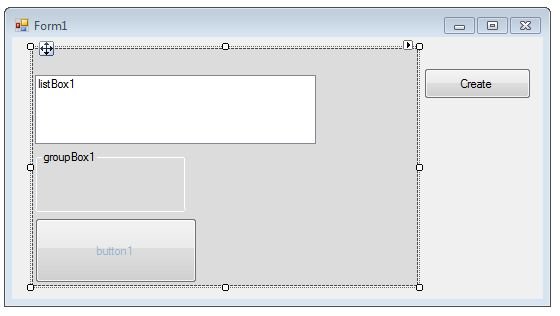
Figure 6
Wrap Contents
The WrapContents property represents if the contents of a FlowLayoutPanel control are wrapped or not. The following code snippet sets the WrapContents property to true.
dynamicFlowLayoutPanel.WrapContents = True
Figure 7 is a FlowLayoutPanel with WrapContents set to false.
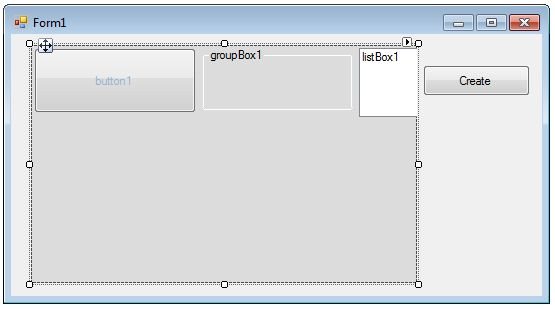
Figure 7
Figure 8 is the same FlowLayoutPanel with WrapContents set to true.
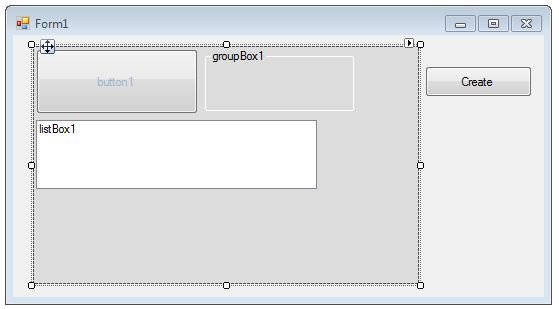
Figure 8
Summary
In this article, we discussed discuss how to create a FlowLayoutPanel control in Windows Forms at design-time as well as run-time. After that, we saw how to use various properties and methods.