Timer Control in VB.NET
The Timer control allows you to set a time interval to execute an event after that interval continuously. It is useful when you want to execute certain applications after a certain interval.
The Timer control allows you to set a time interval to execute an event after that interval continuously. It is useful when you want to execute certain applications after a certain interval. Say you want to create a backup of your data processing in every hour. You can make a routine which will take the backup and call that routine on Timer's event and set timer interval for an hour.
Using timer control is pretty simple. To test the control, I'm going to create a Windows Application. I also add two button controls to the form and change their text to Start and Stop as you can see from the following Figure.
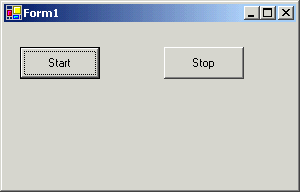
In this application, I'm going to create a text file mcb.txt in your C:\temp directory and start writing the time after 5 seconds interval. Stop button stops the timer and Start again start the timer.
Now you can drag a timer control from the toolbox to the form. You can set timer properties from the IDE as well as programmatically. To set the timer properties, right click on the timer control and change Interval property. As you can see from the Figure, I put 5000 milliseconds (5 seconds). 1 sec = 1000 milliseconds (In case your mathematics is poor as mine
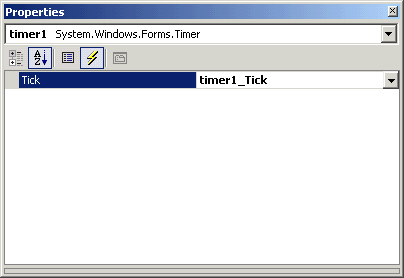
Now click on the Events button and write event for the timer click as you can see from the following figure.
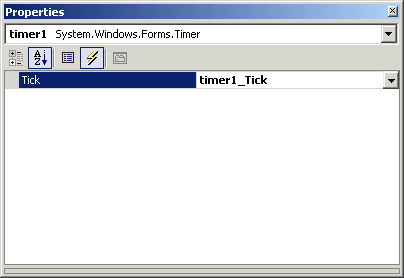
Now I add a FileStream and a StreamWriter object in the beginning of the class. As you can see from the following code, FileStream class creates a mcb.txt file and StreamWriter will be used to write to the file.
private shared fs as new FileStream(@"c:\temp\mcb.txt" , FileMode.OpenOrCreate, FileAccess.Write)
Private Shared m_streamWriter As New StreamWriter(fs)
Now write the following code on the Form Load event:
Private Sub Form1_Load(ByVal sender As Object, ByVal e As System.EventArgs)
' Write to the file using StreamWriter class
m_streamWriter.BaseStream.Seek(0, SeekOrigin.End)
m_streamWriter.Write(" File Write Operation Starts : ")
m_streamWriter.WriteLine("{0} {1}", DateTime.Now.ToLongTimeString(), DateTime.Now.ToLongDateString())
m_streamWriter.WriteLine(" ===================================== " + ControlChars.Lf)
m_streamWriter.Flush()
End Sub 'Form1_Load
As you can see from the above code, this code writes some lines to the file.
Now write code on the start and stop button click handlers. As you can see from the following code, the Start button click sets timer's Enabled property as true. Setting timer's Enabled property starts timer to execute the timer event. I set Enabled property as false on the Stop button click event handler, which stops executing the timer tick event.
Private Sub button1_Click(ByVal sender As Object, ByVal e As System.EventArgs)timer1.Enabled = True
End Sub 'button1_Click
Private Sub button2_Click(ByVal sender As Object, ByVal e As System.EventArgs)timer1.Enabled = False
End Sub 'button2_Click
Now last step is to write timer's tick event to write current time to the text file. Write the following code on your timer event.
Private Sub timer1_Tick(ByVal sender As Object, ByVal e As System.EventArgs)m_streamWriter.WriteLine("{0} {1}", DateTime.Now.ToLongTimeString(), DateTime.Now.ToLongDateString())
m_streamWriter.Flush()
End Sub 'timer1_Tick
Now use Start and Stop buttons to start and stop the timer. The output mcb.txt file looks like the following figure.
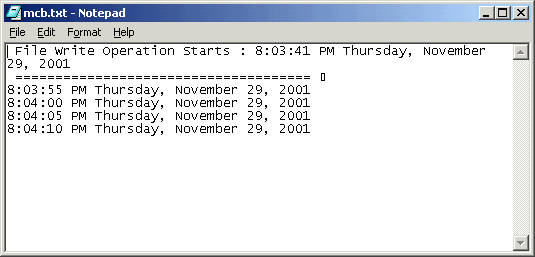
Using Timer control Programmatically
If you don't have Visual Studio .NET, you can also write the same sample. Just following these steps.
Creating an instance of Timer
The Timer class's constructor is used to create a timer object. The constructor is overloaded.
Public Timer()
Public Timer(double) Sets the interval property to the specified.
Here is how to create a Timer with 5 seconds interval.
Timer myTimer = new Timer(500);
Here are some useful members of the Timer class
Tick |
This event occurs when the Interval has elapsed. |
Start |
Starts raising the Tick event by setting Enabled to true. |
Stop |
Stops raising the Tick event by setting Enabled to false. |
Close |
Releases the resources used by the Timer. |
AutoReset |
Indicates whether the Timer raises the Tick event each time the specified Interval has elapsed or whether the Tick event is raised only once after the first interval has elapsed. |
Interval |
Indicates the interval on which to raise the Tick event. |
Enabled |
Indicates whether the Timer raises the Tick event. |
How to use Timer class to raise an event after certain interval?
timer1.Interval = 5000
timer1.Enabled = true
addhandler timer1.Tick,addressof OnTimerEvent
Write the event handler
This event will be executed after every 5 secs.
Public Shared Sub OnTimerEvent(ByVal [source] As Object, ByVal e As EventArgs)m_streamWriter.WriteLine("{0} {1}", DateTime.Now.ToLongTimeString(), DateTime.Now.ToLongDateString())
m_streamWriter.Flush()
End Sub 'OnTimerEvent
You use Stop and Close events to stop the timer and release the resources. See attached project for more details.