TableLayoutPanel control represents a panel that dynamically lays out its contents in a table format. You want to use a TableLayoutPanel in complex and sophisticated applications where you need to create dynamic layouts.
In this article, I will demonstrate how to create and use a TableLayoutPanel control in a Windows Forms application.
Creating a TableLayoutPanel
We can create a TableLayoutPanel control using the Forms designer at design-time or using the TableLayoutPanel class in code at run-time.
Design-time
To create a TableLayoutPanel control at design-time, you simply drag and drop a TableLayoutPanel control from Toolbox onto a Form in Visual Studio. After you drag and drop a TableLayoutPanel on a Form, the TableLayoutPanel looks like Figure 1. Once a TableLayoutPanel is on the Form, you can move it around and resize it using mouse and set its properties and events.
Figure 1
If you click on little handle of TableLayoutPanel, you will see options to add, remove, and edit rows and columns of the panel. Once rows and columns are added to a TableLayoutPanel, we can drag and drop child controls to these cells to manage the layouts.
Run-time
Creating a TableLayoutPanel control at run-time is merely a work of creating an instance of TableLayoutPanel class, set its properties and adds TableLayoutPanel class to the Form controls.
First step to create a dynamic TableLayoutPanel is to create an instance of TableLayoutPanel class. The following code snippet creates a TableLayoutPanel control object.
Dim dynamicTableLayoutPanel As New TableLayoutPanel()
In the next step, you may set properties of a TableLayoutPanel control. The following code snippet sets location, size and Name properties of a TableLayoutPanel.
dynamicTableLayoutPanel.Location = New System.Drawing.Point(26, 12)
dynamicTableLayoutPanel.Name = "TableLayoutPanel1"
dynamicTableLayoutPanel.Size = New System.Drawing.Size(228, 200)
dynamicTableLayoutPanel.BackColor = Color.LightBlue
Once the TableLayoutPanel control is ready with its properties, the next step is to add the TableLayoutPanel to a Form. To do so, we use Form.Controls.Add method that adds TableLayoutPanel control to the Form controls and displays on the Form based on the location and size of the control. The following code snippet adds a TableLayoutPanel control to the current Form.
Controls.Add(dynamicTableLayoutPanel)
Adding Controls to a TableLayoutPanel
You can add controls to a TableLayoutPanel by dragging and dropping control to the TableLayoutPanel. We can add controls to a TableLayoutPanel at run-time by using its Add method.
ColumnStyle and RowStyle properties are used to specify number of columns and rows in a TableLayoutPanel. Add method is used to add a row and column to RowStyle and ColumnStyle respectively.
dynamicTableLayoutPanel.ColumnStyles.Add(New ColumnStyle(SizeType.Percent, 30.0F))
dynamicTableLayoutPanel.RowStyles.Add(New RowStyle(SizeType.Absolute, 52.0F))
We can specify the position of a cell (row number and column number) in TableLayoutPanel.Controls.Add() method as following.
dynamicTableLayoutPanel.Controls.Add(textBox1, 0, 0)
The following code snippet creates a TableLayoutPanel, creates a TextBox and a CheckBox and adds these two controls to a TableLayoutPanel.
Private Sub Form1_Load(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles MyBase.Load
Dim dynamicTableLayoutPanel As New TableLayoutPanel()
dynamicTableLayoutPanel.Location = New System.Drawing.Point(26, 12)
dynamicTableLayoutPanel.Name = "TableLayoutPanel1"
dynamicTableLayoutPanel.Size = New System.Drawing.Size(228, 200)
dynamicTableLayoutPanel.BackColor = Color.LightBlue
dynamicTableLayoutPanel.ColumnCount = 3
dynamicTableLayoutPanel.RowCount = 5
dynamicTableLayoutPanel.ColumnStyles.Add(New ColumnStyle(SizeType.Percent, 30.0F))
dynamicTableLayoutPanel.ColumnStyles.Add(New ColumnStyle(SizeType.Percent, 30.0F))
dynamicTableLayoutPanel.ColumnStyles.Add(New ColumnStyle(SizeType.Percent, 40.0F))
dynamicTableLayoutPanel.RowStyles.Add(New RowStyle(SizeType.Absolute, 52.0F))
dynamicTableLayoutPanel.RowStyles.Add(New RowStyle(SizeType.Absolute, 44.0F))
dynamicTableLayoutPanel.RowStyles.Add(New RowStyle(SizeType.Absolute, 44.0F))
dynamicTableLayoutPanel.RowStyles.Add(New RowStyle(SizeType.Absolute, 38.0F))
dynamicTableLayoutPanel.RowStyles.Add(New RowStyle(SizeType.Absolute, 8.0F))
Dim textBox1 As New TextBox()
textBox1.Location = New Point(10, 10)
textBox1.Text = "I am a TextBox5"
textBox1.Size = New Size(200, 30)
Dim checkBox1 As New CheckBox()
checkBox1.Location = New Point(10, 50)
checkBox1.Text = "Check Me"
checkBox1.Size = New Size(200, 30)
dynamicTableLayoutPanel.Controls.Add(textBox1, 0, 0)
dynamicTableLayoutPanel.Controls.Add(checkBox1, 0, 1)
Controls.Add(dynamicTableLayoutPanel)
End Sub
The output looks like Figure 2.
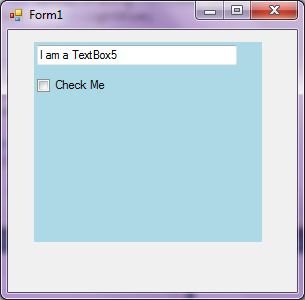
Figure 2
Show and Hide a TableLayoutPanel
I have seen in many applications when you want to show and hide a group of controls on a Form based on some condition. That is where a TableLayoutPanel is useful. Instead of show and hide individual controls, we can group controls that we want to show and hide and place them on two different TableLayoutPanels and show and hide the TableLayoutPanels. To show and hide a TableLayoutPanel, we use Visible property.
dynamicTableLayoutPanel.Visible = False
Summary
In this article, we discussed how to use a TableLayoutPanel control in a Windows Forms application.