A GroupBox control is a container control that is used to place Windows Forms child controls in a group. The purpose of a GroupBox is to define user interfaces where we can categories related controls in a group.
In this article, I will discuss how to create a GroupBox control in Windows Forms at design-time as well as run-time. After that, I will discuss various properties and methods available for the GroupBox control.
Creating a GroupBox
We can create a GroupBox control using a Forms designer at design-time or using the GroupBox class in code at run-time (also known as dynamically).
To create a GroupBox control at design-time, you simply drag and drop a GroupBox control from Toolbox to a Form in Visual Studio. After you drag and drop a GroupBox on a Form, the GroupBox looks like Figure 1. Once a GroupBox is on the Form, you can move it around and resize it using mouse and set its properties and events.
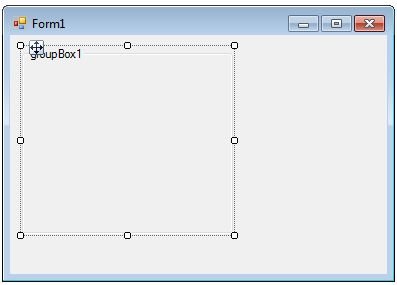
Figure 1
Creating a GroupBox control at run-time is merely a work of creating an instance of GroupBox class, set its properties and add GroupBox class to the Form controls.
First step to create a dynamic GroupBox is to create an instance of GroupBox class. The following code snippet creates a GroupBox control object.
Dim authorGroup As New GroupBox
In the next step, you may set properties of a GroupBox control. The following code snippet sets background color, foreground color, Text, Name, and Font properties of a GroupBox.
authorGroup.Name = "GroupBox1"
authorGroup.BackColor = Color.LightBlue
authorGroup.ForeColor = Color.Maroon
authorGroup.Text = "Author Details"
authorGroup.Font = New Font("Georgia", 12)
Once a GroupBox control is ready with its properties, next step is to add the GroupBox control to the Form. To do so, we use Form.Controls.Add method. The following code snippet adds a GroupBox control to the current Form.
Me.Controls.Add(authorGroup)
Setting GroupBox Properties
After you place a GroupBox control on a Form, the next step is to set properties.
The easiest way to set properties is from the Properties Window. You can open Properties window by pressing F4 or right click on a control and select Properties menu item. The Properties window looks like Figure 2.
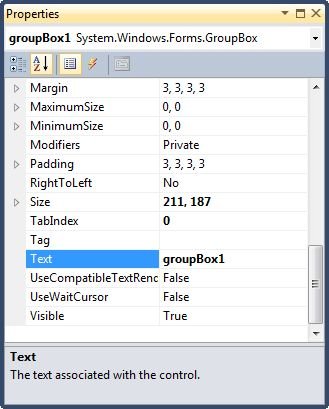
Figure 2
Location, Height, Width, and Size
The Location property takes a Point that specifies the starting position of the GroupBox on a Form. The Size property specifies the size of the control. We can also use Width and Height property instead of Size property. The following code snippet sets Location, Width, and Height properties of a GroupBox control.
authorGroup.Location = New Point(10, 10)
authorGroup.Width = 250
authorGroup.Height = 200
Background and Foreground
BackColor and ForeColor properties are used to set background and foreground color of a GroupBox respectively. If you click on these properties in Properties window, the Color Dialog pops up.
Alternatively, you can set background and foreground colors at run-time. The following code snippet sets BackColor and ForeColor properties.
authorGroup.BackColor = Color.LightBlue
authorGroup.ForeColor = Color.Maroon
Name
Name property represents a unique name of a GroupBox control. It is used to access the control in the code. The following code snippet sets and gets the name and text of a GroupBox control.
authorGroup.Name = "GroupBox1"
Text
Text property of a GroupBox represents the header text of a GroupBox control. The following code snippet sets the header of a GroupBox control.
authorGroup.Text = "Author Details"
Font
Font property represents the font of header text of a GroupBox control. If you click on the Font property in Properties window, you will see Font name, size and other font options. The following code snippet sets Font property at run-time.
dynamicGroupBox.Font = New Font("Georgia", 12)
Summary
A GroupBox control is a container control used to group similar controls. In this article, we discussed discuss how to create a GroupBox control in Windows Forms at design-time as well as run-time. After that, we saw how to use various properties and methods.