The MenuStrip class is the foundation of menus functionality in Windows Forms. If you have worked with menus in .NET 1.0 and 2.0, you must be familiar with the MainMenu control. In .NET 3.5 and 4.0, the MainMenu control is replaced with the MenuStrip control.
Creating a MenuStrip
We can create a MenuStrip control using a Forms designer at design-time or using the MenuStrip class in code at run-time or dynamically.
To create a MenuStrip control at design-time, you simply drag and drop a MenuStrip control from Toolbox to a Form in Visual Studio. After you drag and drop a MenuStrip on a Form, the MenuStrip1 is added to the Form and looks like Figure 1. Once a MenuStrip is on the Form, you can add menu items and set its properties and events.
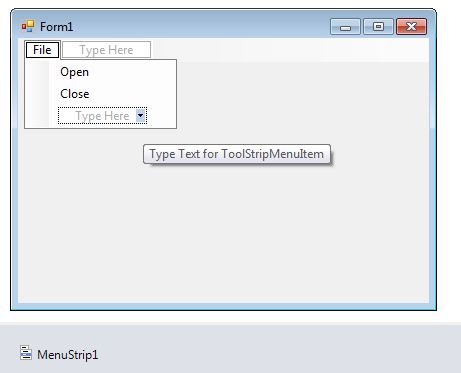
Figure 1
Creating a MenuStrip control at run-time is merely a work of creating an instance of MenuStrip class, set its properties and adds MenuStrip class to the Form controls.
First step to create a dynamic MenuStrip is to create an instance of MenuStrip class. The following code snippet creates a MenuStrip control object.
C# Code:
MenuStrip MainMenu = new MenuStrip();
VB.NET Code:
Dim MainMenu As New MenuStrip()
In the next step, you may set properties of a MenuStrip control. The following code snippet sets background color, foreground color, Text, Name, and Font properties of a MenuStrip.
MainMenu.BackColor = Color.OrangeRed
MainMenu.ForeColor = Color.Black
MainMenu.Text = "File Menu"
MainMenu.Font = New Font("Georgia", 16)
Once the MenuStrip control is ready with its properties, the next step is to add the MenuStrip to a Form. To do so, first we set MainMenuStrip property and then use Form.Controls.Add method that adds MenuStrip control to the Form controls and displays on the Form based on the location and size of the control. The following code snippet adds a MenuStrip control to the current Form.
Me.MainMenuStrip = MainMenu
Controls.Add(MainMenu)
Setting MenuStrip Properties
After you place a MenuStrip control on a Form, the next step is to set properties.
The easiest way to set properties is from the Properties Window. You can open Properties window by pressing F4 or right click on a control and select Properties menu item. The Properties window looks like Figure 2.
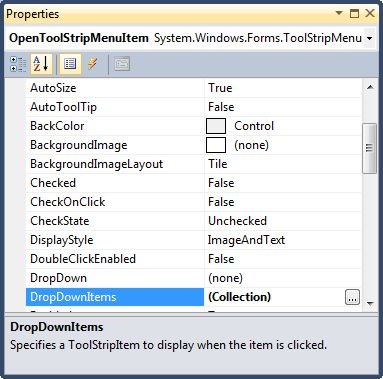
Figure 2
Name
Name property represents a unique name of a MenuStrip control. It is used to access the control in the code. The following code snippet sets and gets the name and text of a MenuStrip control.
MainMenu.Name = "MailMenu"
Positioning a MenuStrip
The Dock property is used to set the position of a MenuStrip. It is of type DockStyle that can have values Top, Bottom, Left, Right, and Fill. The following code snippet sets Location, Width, and Height properties of a MenuStrip control.
MainMenu.Dock = DockStyle.Left
Font
Font property represents the font of text of a MenuStrip control. If you click on the Font property in Properties window, you will see Font name, size and other font options. The following code snippet sets Font property at run-time.
MainMenu.Font = new Font("Georgia", 16)
Background and Foreground
BackColor and ForeColor properties are used to set background and foreground color of a MenuStrip respectively. If you click on these properties in Properties window, the Color Dialog pops up.
Alternatively, you can set background and foreground colors at run-time. The following code snippet sets BackColor and ForeColor properties.
MainMenu.BackColor = System.Drawing.Color.OrangeRed
MainMenu.ForeColor = System.Drawing.Color.Black
The new MenuStrip with background and foreground looks like Figure 3.
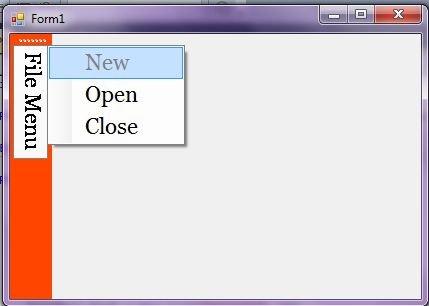
Figure 3
MenuStrip Items
A Menu control is nothing without menu items. The Items property is used to add and work with items in a MenuStrip. We can add items to a MenuStrip at design-time from Properties Window by clicking on Items Collection as you can see in Figure 4.
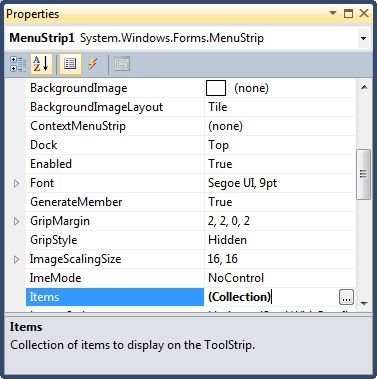
Figure 4
When you click on the Collections, the String Collection Editor window will pop up where you can type strings. Each line added to this collection will become a MenuStrip item. I add four items as you can see from Figure 5.
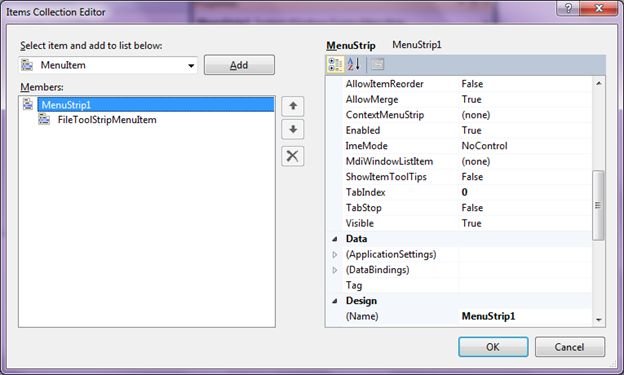
Figure 5
A ToolStripMenuItem represents a menu items. The following code snippet creates a menu item and sets its properties.
Dim FileMenu As New ToolStripMenuItem("File")
FileMenu.BackColor = Color.OrangeRed
FileMenu.ForeColor = Color.Black
FileMenu.Text = "File Menu"
FileMenu.Font = New Font("Georgia", 16)
FileMenu.TextAlign = ContentAlignment.BottomRight
FileMenu.TextDirection = ToolStripTextDirection.Vertical90
FileMenu.ToolTipText = "Click Me"
Once a menu item is created, we can add it to the main menu by using MenuStrip.Items.Add method. The following code snippet adds FileMenu item to the MainMenu.
MainMenu.Items.Add(FileMenu)
Adding Menu Item Click Event Handler
The main purpose of a menu item is to add a click event handler and write code that we need to execute on the menu item click event handler. For example, on File >> New menu item click event handler, we may want to create a new file.
To add an event handler, you go to Events window and double click on Click and other as you can see in Figure 6.
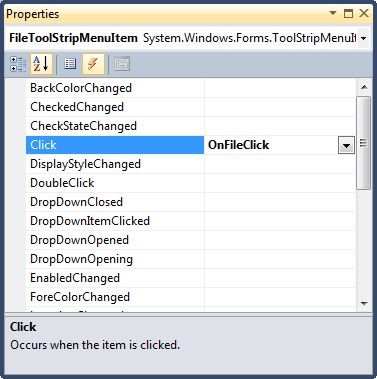
Figure 6
We can also define and implement an event handler dynamically. The following code snippet defines and implements these events and their respective event handlers.
Dim NewMenuItem As New ToolStripMenuItem("New", Nothing, _
New EventHandler(AddressOf NewMenuItemClick))
Private Sub NewMenuItemClick(ByVal sender As Object, ByVal e As EventArgs)
MessageBox.Show("New menu item clicked!")
End Sub
Summary
In this article, we discussed discuss how to create menus using the MenuStrip control. First we discussed how to create menus at design-time and run-time. After that we saw, how to set menus properties and click event handlers.